@BRT.RegData()
Retrieves the registrations, registrants and questions from a specified Reg form.
The @BRT.RegData() Helper retrieves registration data from a specified form. The required formId: parameter sets the numeric Id of the form to be retrieved. If the optional template: parameter is used the registration data is accessed through the EventRegData class in the RegDataHelperExtensions namespace. This class returns the following:
Registration Class
- Id - (integer)
- RegisterDate - (datetime)
- Cancelled - (bool)
- ContactName - (string)
- ContactEmail - (string)
- Total - (decimal)
- Paid - (decimal)
- Registrants - (list of Registrant)
- RegistrationPayments - (Payments)
Registrant Class
- Id - (integer)
- FirstName - (string)
- LastName - (string)
- PreferredName - (string)
- Address1 - (string)
- Address2 - (string)
- City - (string)
- State - (string)
- Zip - (string)
- Phone - (string)
- Email - (string)
- Answers - (list of RegistrantAnswers)
Payments Class
- Id - (integer)
- PayPal - (integer, nullable)
- Check - (integer, nullable)
- Cash - (integer, nullable)
- Adjustment - (integer, nullable)
- NotRequired - (integer, nullable)
- Refund - (integer, nullable)
- Voucher - (integer, nullable)
- PPBraintree - (integer, nullable)
RegistrantAnswer Class
- RegistrantId - (integer, nullable)
- QuestionId - (integer)
- Answer - (string)
- AnswerReporting - (string)
- QuestionLabel - (string)
Question Class
- Id - (integer)
- Label - (string)
- MultiAnswer - (boolean)
- Choices - (list of Choice)
Choice Class
- Id - (integer)
- Choice - (string)
- ReportId - (string)
Answer Class
- RegistrantId - (integer, nullable)
- QuestionId - (integer)
- AnswerInt - (integer, nullable)
- AnswerMultiLine - (string)
- AnswerSingleLine - (string)
Templated Example
@BRT.RegData(1111, template: @<div> <h3>Custom</h3> <table> <tr> <th>Date</th> <th>Contact</th> <th>Leaders</th> @foreach(RegDataHelperExtensions.EventRegData.Question q in item.Questions){ <th class="regq">@q.Label</th> } <th class="regq">Total for Registration</th> <th class="regq">Paid for Registration</th> <th class="regq">Owed for Registration</th> <th>Cancelled</th> </tr> @foreach(RegDataHelperExtensions.EventRegData.Registration reg in item.Registrations){ <tr> <td>@reg.RegisterDate.ToCustomerDateTime().ToShortDateString()</td> @foreach(RegDataHelperExtensions.EventRegData.Registrant registrant in reg.Registrants){ if(reg.Registrants.IndexOf(registrant) == 0){ <td> <div>@(reg.ContactName == ""? registrant.FirstName + " " + registrant.LastName:reg.ContactName)</div> <div>@(reg.ContactEmail == ""? registrant.Email:reg.ContactEmail)</div> </td> } <td> <div class="emphme">@registrant.FirstName @registrant.PreferredName @registrant.LastName</div> <div>@registrant.Address1</div> <div>@registrant.Address2</div> <div>@registrant.City</div> <div>@registrant.State</div> <div>@registrant.Zip</div> <div>@registrant.Phone</div> <div>@registrant.Email</div> </td> } @foreach(RegDataHelperExtensions.EventRegData.Registrant registrant in reg.Registrants){ if(reg.Registrants.IndexOf(registrant) == 0){ foreach(RegDataHelperExtensions.EventRegData.RegistrantAnswer a in registrant.Answers){ <td class="regq">@a.Answer</td> } break; } } <td class="regq">@reg.Total.ToString("f2")</td> <td class="regq">@reg.Paid.ToString("f2")</td> <td class="regq">@((reg.Total - reg.Paid).ToString("f2"))</td> <td>@(reg.Cancelled? "Yes":"")</td> </tr> } </table> </div>)
Required Parameters
formId: The numeric Id of a Data or Registration Form
Example: formId:250
formId: The numeric Id of a Data or Registration Form
Example: formId:250
Each Data and Registration Form is identified by unique numeric ID when saved for the first time.
Data Form IDs
When a Data Form is opened in the Web Console, this numeric Id is revealed in the sample @BRT.DataLink() helper that is generated by the system.
Registration Form ID
The Registration Form ID is revealed in the URL string when a Registration Form is opened in the Web Console ( or in a browser's status bar when pointing to to link to a Registration Form.
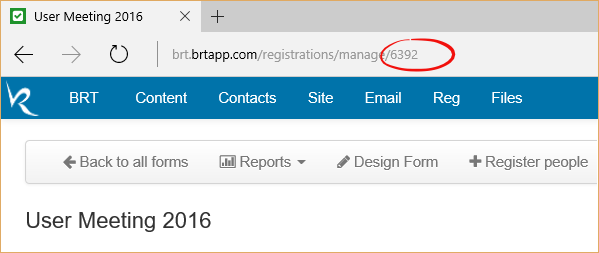
Optional Parameters
pageSize: The number of records to return per page
Example: pageSize:10
pageSize: The number of records to return per page
Example: pageSize:10
Indicates the number Engine Records retrieve and display.
template: Applies a Razor template to data elements retrieved by the Helper
Example: template:
Example: template:
@<div> <h4>Meet our Authors!</h4> @foreach(EngineRecord c in item) { <p><a href="/[email protected]("Name")">@c.GetString("Name") </a></p> } </div>)
The template: parameter is always an optional parameter but virtually all Helpers that create EngineRecord items use either 'template:' or 'itemTemplate:' to style and present item content.
Syntax is:
template:
@<div>
Any valid HTML and Razor code
</div>)